NextJS Using JSON Placeholder Posts
by Keith Rowles • 28/11/2023React
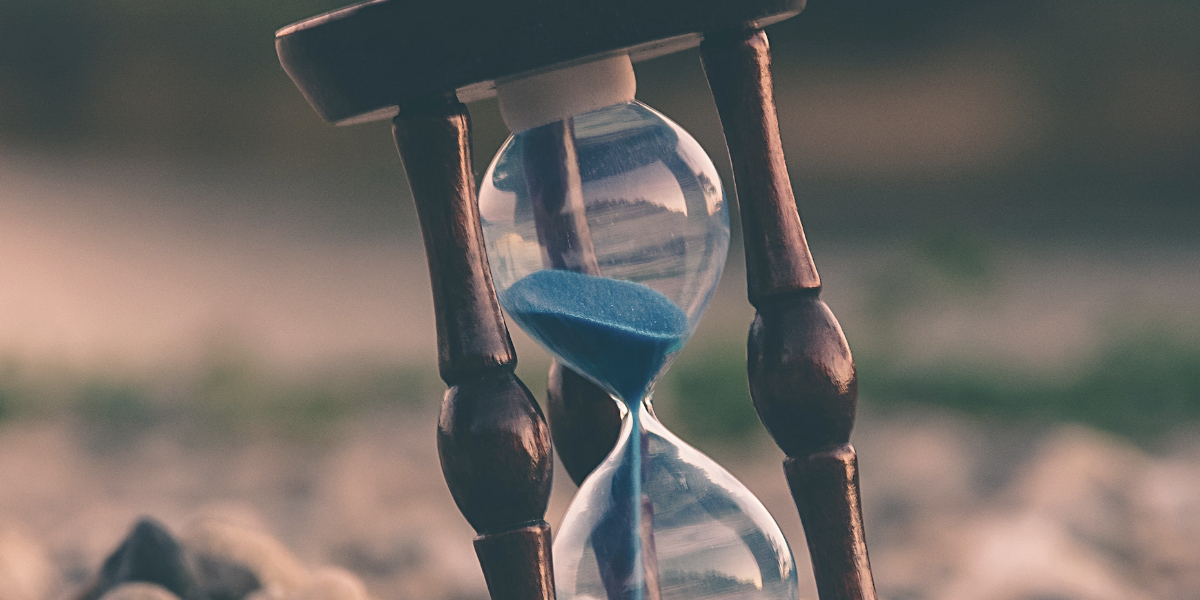
Summary
A simple website displaying posts from the JSON placeholder website using the Next JS framework.
Instead of using all 100 dummy posts I limited the query to 10 posts.
https://jsonplaceholder.typicode.com/posts?_limit=10
The demo is using new version of React but older version of Next.
"dependencies": {
"next": "12.3.1",
"react": "18.2.0",
"react-dom": "18.2.0"
}
I have kept most of the default styles from the default global.css file.
I have other posts using React version 18 and Next JS version 13.
This website demonstrates some features from Next JS:
- Next Head
- Next Link
- Page Routing - home page, about us page and article listing page.
- Components eg. Footer, Layout, Nav, Article List
- getStaticProps
- getStaticPaths
- API endpoint
- CSS Modules
Tech and Tools
- JavaScript
- CSS
- HTML
- Next JS
- GitHub
- Vercel
Sample Code
index.js
import ArticleList from '../components/ArticleList';
export default function Home({ articles }) {
// console.log(articles);
return (
<div>
<ArticleList articles={articles} />
</div>
);
}
export const getStaticProps = async () => {
const res = await fetch(
`https://jsonplaceholder.typicode.com/posts?_limit=10`
);
const articles = await res.json();
return {
props: {
articles,
},
};
};
Nav.js
import Link from 'next/link';
import navStyles from '../styles/Nav.module.css';
const Nav = () => {
return (
<nav className={navStyles.nav}>
<ul>
<li>
<Link href="/">Home</Link>
</li>
<li>
<Link href="/about">About</Link>
</li>
</ul>
</nav>
);
};
export default Nav;
Meta.js
import Head from 'next/head';
const Meta = ({ title, keywords, description }) => {
return (
<Head>
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="keywords" content={keywords} />
<meta name="description" content={description} />
<meta charSet="utf-8" />
<link rel="icon" href="/favicon.ico" />
<title>{title}</title>
</Head>
);
};
Meta.defaultProps = {
title: 'WebDev News',
keywords: 'web development, programming',
description: 'Get the latest news in web dev.',
};
export default Meta;
Article.module.css
.grid {
display: flex;
align-items: center;
justify-content: center;
flex-wrap: wrap;
max-width: 800px;
margin-top: 3rem;
}
.card {
margin: 1rem;
flex-basis: 45%;
padding: 1.5rem;
text-align: left;
color: inherit;
text-decoration: none;
border: 1px solid #eaeaea;
border-radius: 10px;
transition: color 0.15s ease, border-color 0.15s ease;
}
.card:hover,
.card:focus,
.card:active {
color: #0070f3;
border-color: #0070f3;
}
.card h3 {
margin: 0 0 1rem 0;
font-size: 1.5rem;
}
.card p {
margin: 0;
font-size: 1.25rem;
line-height: 1.5;
}
.logo {
height: 1em;
}
@media (max-width: 600px) {
.grid {
width: 100%;
flex-direction: column;
}
}
Demo
Open demo on Vercel.
Link to DemoRepo
Open GitHub Repository to view full source code.
Link to Repo