Parsley JS Validation
by Keith Rowles • 01/10/2023JavaScript
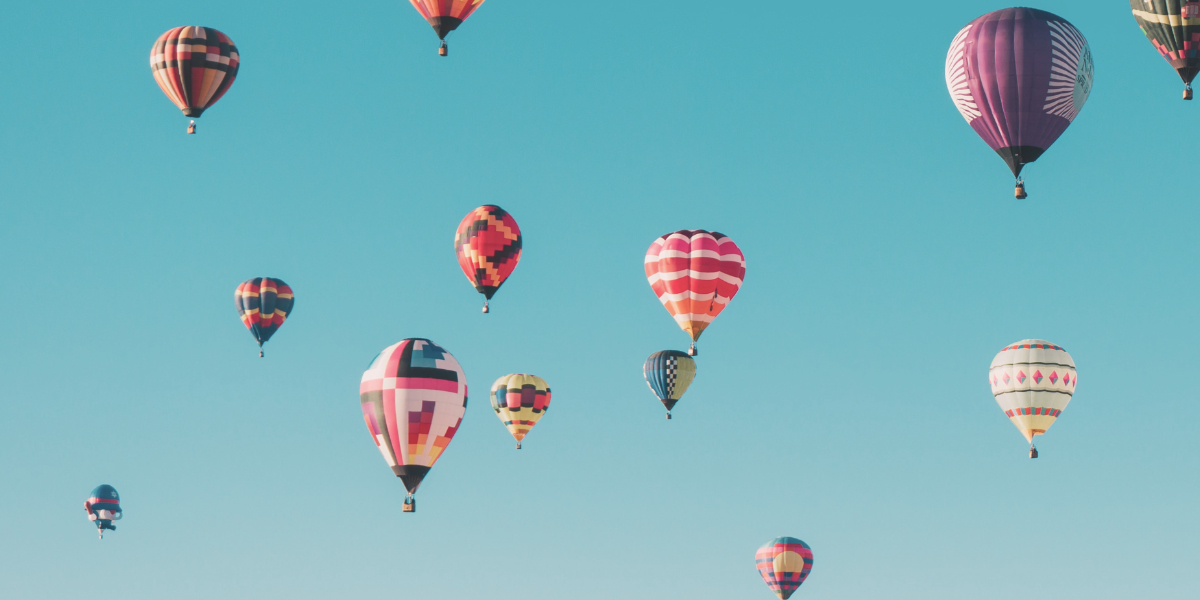
Summary
A simple demonstration on how to use the parsley js validation library to validate a website form.
Great tool!
HTML code
<div class="container mt-5">
<div class="bs-callout bs-callout-warning d-none">
<h4>Oh snap!</h4>
<p>This form seems to be invalid :(</p>
</div>
<div class="bs-callout bs-callout-info d-none">
<h4>Yay!</h4>
<p>Everything seems to be ok :)</p>
</div>
<form id="demo-form" data-parsley-validate="">
<!-- Name -->
<div class="mb-3">
<label for="fullname" class="form-label">Full Name <span class="required">*</span> :</label>
<input type="text" class="form-control" name="fullname" required="" minlength="5">
</div>
<!-- Full Name -->
<div class="row mb-3">
<div class="col">
<label for="firstname" class="form-label">First Name <span class="required">*</span> :</label>
<input type="text" class="form-control" name="firstname" required="" minlength="3">
</div>
<div class="col">
<label for="lastname" class="form-label">Last Name <span class="required">*</span> :</label>
<input type="text" class="form-control" name="lastname" required="" minlength="3">
</div>
</div>
<!-- Email Address -->
<div class="mb-3">
<label for="email" class="form-label">Email <span class="required">*</span> :</label>
<input type="email" class="form-control" name="email" data-parsley-trigger="change" required="">
</div>
<!-- Website URL -->
<div class="mb-3">
<label for="website" class="form-label">Website <span class="required">*</span> :</label>
<input type="url" class="form-control" name="website" data-parsley-trigger="change" required="">
</div>
<!-- Address -->
<div class="row mb-3">
<div class="col-md-6">
<label for="city" class="form-label">City <span class="required">*</span> :</label>
<input type="text" class="form-control" name="city" data-parsley-trigger="change" required="">
</div>
<div class="col-md-4">
<label for="state" class="form-label">State <span class="required">*</span> :</label>
<select id="state" class="form-select" required>
<option value="">Choose...</option>
<option value="NSW">New South Wales</option>
<option value="QLD">Queensland</option>
<option value="NT">Northern Territory</option>
</select>
</div>
<div class="col-md-2">
<label for="postcode" class="form-label">Post Code <span class="required">*</span> :</label>
<input type="text" class="form-control" name="postcode" required="" data-parsley-type="number" data-parsley-minlength="4" data-parsley-maxlength="4">
</div>
</div>
<!-- Radio Buttons -->
<div class="mb-3">
<label for="contactMethod" class="form-check-label">Preferred Contact Method <span class="required">*</span>:</label>
<div class="form-check">
Email: <input type="radio" name="contactMethod" id="contactMethodEmail" value="Email" required="" class="form-check-input">
</div>
<div class="form-check">
Phone: <input type="radio" name="contactMethod" id="contactMethodPhone" value="Phone" class="form-check-input">
</div>
</div>
<!-- Checkboxes -->
<div class="mb-3">
<label for="hobbies">Hobbies (Optional, but 2 minimum):</label>
<div class="form-check">
<input type="checkbox" name="hobbies[]" id="hobby1" value="ski" data-parsley-mincheck="2" class="form-check-input">
<label class="form-check-label" for="hobby1">Skiing</label>
</div>
<div class="form-check">
<input type="checkbox" name="hobbies[]" id="hobby2" value="run" class="form-check-input">
<label class="form-check-label" for="hobby2">Running</label>
</div>
<div class="form-check">
<input type="checkbox" name="hobbies[]" id="hobby3" value="eat" class="form-check-input">
<label class="form-check-label" for="hobby3">Eating</label>
</div>
<div class="form-check">
<input type="checkbox" name="hobbies[]" id="hobby4" value="sleep" class="form-check-input">
<label class="form-check-label" for="hobby4">Sleeping</label>
</div>
<div class="form-check">
<input type="checkbox" name="hobbies[]" id="hobby5" value="read" class="form-check-input">
<label class="form-check-label" for="hobby5">Reading</label>
</div>
<div class="form-check">
<input type="checkbox" name="hobbies[]" id="hobby6" value="code" class="form-check-input">
<label class="form-check-label" for="hobby6">Coding</label>
</div>
</div>
<!-- Select Box -->
<div class="mb-3">
<label for="heard" class="form-label">Heard about us via <span class="required">*</span>:</label>
<select class="form-select" id="heard" required="">
<option value="">Choose..</option>
<option value="press">Press</option>
<option value="net">Internet</option>
<option value="mouth">Word of mouth</option>
<option value="other">Other..</option>
</select>
</div>
<!-- Text Area -->
<div class="mb-3">
<label for="message" class="form-label">Message (20 chars min, 100 max) :</label>
<textarea id="message" class="form-control" name="message" data-parsley-trigger="keyup" data-parsley-minlength="20" data-parsley-maxlength="100" data-parsley-minlength-message="Come on! You need to enter at least a 20 character comment.." data-parsley-validation-threshold="10"></textarea>
</div>
<!-- Button -->
<br>
<input type="submit" class="btn btn-primary" value="validate" role="button">
</div>
JS Code
Using JQuery.
$(function () {
$('#demo-form')
.parsley()
.on('field:validated', function () {
var ok = $('.parsley-error').length === 0;
$('.bs-callout-info').toggleClass('d-none', !ok);
$('.bs-callout-warning').toggleClass('d-none', ok);
})
.on('form:submit', function () {
return false; // Don't submit form for this demo
});
});
Demonstration
View demonstration in CodePen.
Link to DemoParsley Website
View the library.
Go To Website