Pricing Table Using CSS Variables
by Keith Rowles • 22/10/2023CSS
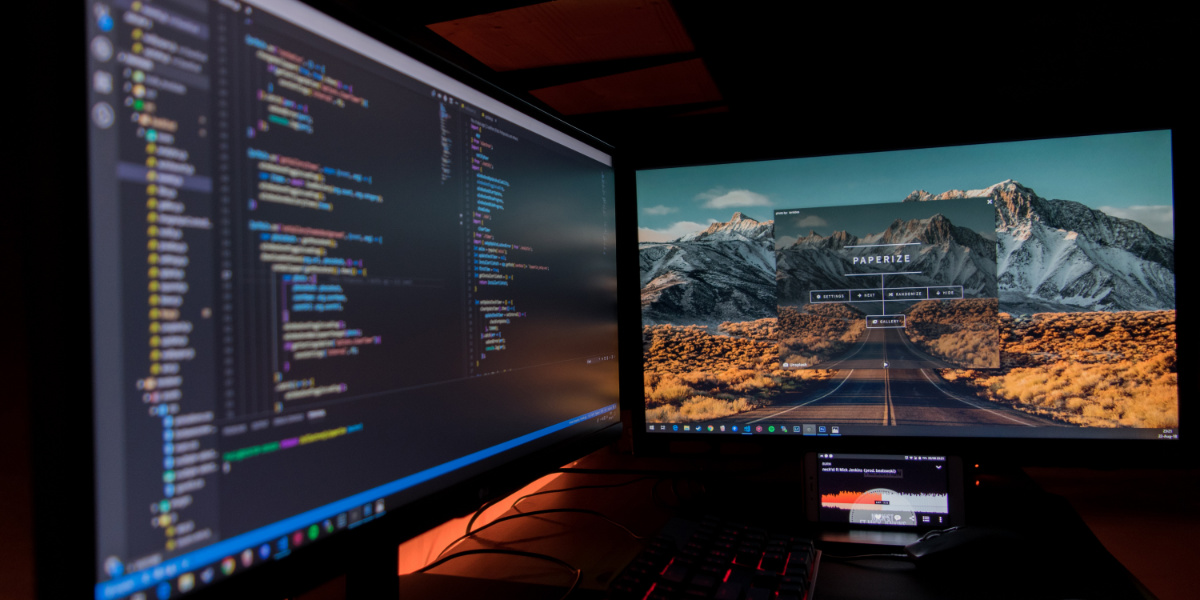
Summary
Here we create a pricing component showing available plans plus the best deal using css variables.
There are 3 plans placed into cards.
We have the following plans:
- Basic
- Best Value
- Super
Setup
Lets import a Google font.
@import url('https://fonts.googleapis.com/css?family=Fira+Sans:300,600,800');
Here are the css variables. We are declaring the font face, font weight, font size, primary and secondary colours, margin and padding, shadow and border radius.
:root {
--ff: 'Fira Sans', sans-serif;
--fw-n: 300;
--fw-m: 600;
--fw-b: 800;
--fs-2: 5rem;
--fs-p: 1rem;
--primary-clr: #225560;
--secondary-clr: #36213e;
--secondary-clr-light: #554971;
--accent-clr: #ef6461;
--spacer: 1rem;
--spacer-md: calc(var(--spacer) * 2);
--spacer-lg: calc(var(--spacer) * 3);
--shadow: 0 0 1em rgba(0, 0, 0, 0.25);
--br: 5px;
}
We will set the box sizing to border box.
* {
box-sizing: border-box;
}
Style the base html elements.
body {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
font-family: var(--ff);
}
h2 {
font-size: var(--fs-2);
font-weight: var(--fw-l);
margin: 0 0 0.5em;
}
p {
margin: 0 0 var(--spacer);
}
strong {
font-weight: var(--fw-m);
}
Style the buttons.
.button {
display: inline-block;
background: var(--primary-clr);
color: white;
text-decoration: none;
padding: 1em 1.5em;
border-radius: var(--br);
font-weight: var(--fw-b);
text-transform: uppercase;
letter-spacing: 2px;
font-size: 0.8rem;
}
.button--card {
margin-top: var(--spacer);
}
Style the main page container and the price grid.
.container {
width: 90%;
max-width: 1200px;
margin: 0 auto;
position: relative;
}
.pricing__grid {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-column-gap: var(--spacer-md);
}
And now for the cards.
.card {
border-radius: var(--br);
overflow: hidden;
box-shadow: var(--shadow);
}
.card--important {
--primary-clr: var(--accent-clr);
}
.card__title {
background: var(--primary-clr);
color: rgba(255, 255, 255, 0.7);
margin: 0;
padding: var(--spacer) 0;
font-weight: var(--fw-m);
letter-spacing: 2px;
text-align: center;
font-size: 0.8rem;
text-transform: uppercase;
}
.card__price {
color: var(--primary-clr);
padding: 1em var(--spacer-lg);
margin: 0;
font-size: 2em;
font-weight: var(--fw-b);
}
.card__price span {
font-size: 0.5em;
font-weight: var(--fw-n);
}
.card__body {
padding: 0 var(--spacer-lg) 2em;
}
Here is the html.
<section class="pricing">
<div class="container">
<h2>Pricing</h2>
<div class="pricing__grid">
<div class="card">
<h3 class="card__title">Basic</h3>
<p class="card__price">$29<span>/month</span></p>
<div class="card__body">
<p><strong>Free</strong> sales point one</p>
<p><strong>Unlimited</strong> sales point two</p>
<p><strong>Really good</strong> sales point three</p>
<p><strong>Unlimited</strong> sales point four</p>
<a href="#" class="button button--card">Buy now</a>
</div>
</div>
<div class="card card--important">
<h3 class="card__title">Best Value</h3>
<p class="card__price">$49<span>/month</span></p>
<div class="card__body">
<p><strong>Free</strong> sales point one</p>
<p><strong>Unlimited</strong> sales point two</p>
<p><strong>Really good</strong> sales point three</p>
<p><strong>Unlimited</strong> sales point four</p>
<a href="#" class="button button--card">Buy now</a>
</div>
</div>
<div class="card">
<h3 class="card__title">Super</h3>
<p class="card__price">$109<span>/month</span></p>
<div class="card__body">
<p><strong>Free</strong> sales point one</p>
<p><strong>Unlimited</strong> sales point two</p>
<p><strong>Really good</strong> sales point three</p>
<p><strong>Unlimited</strong> sales point four</p>
<a href="#" class="button button--card">Buy now</a>
</div>
</div>
</div>
</div>
</section>
Tech and Tools
- CSS
- HTML
- Code Pen
Demo
Open demo on Code Pen.
Link to Demo