Sticky Notes JavaScript App With Local Storage
by Keith Rowles • 25/12/2023JavaScript
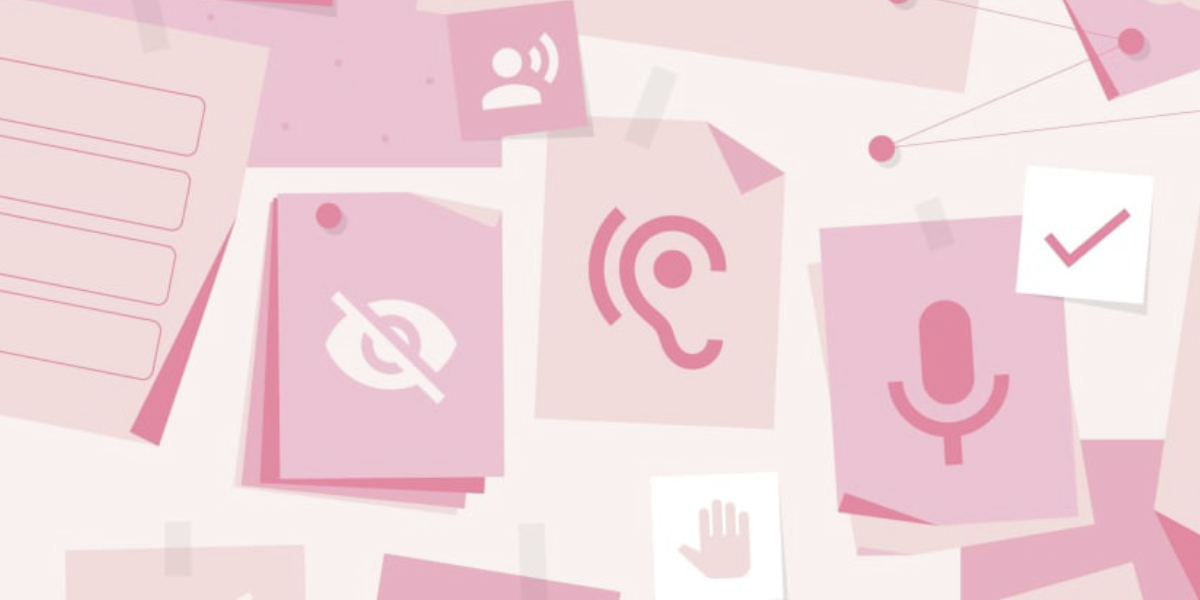
Summary
Fun sticky notes application built with vanilla javascript and using local storage to store your notes.
Tech
- html
- css
- javascript
- js fiddle
CSS
body {
margin: 0;
background-color: darkcyan;
}
button {
padding: 0;
margin: 0;
}
#app {
display: grid;
grid-template-columns: repeat(auto-fill, 200px);
padding: 24px;
gap: 24px;
}
.note {
height: 200px;
box-sizing: border-box;
padding: 16px;
border: none;
border-radius: 10px;
box-shadow: 0 0 7px rgba(0, 0, 0, 0.25);
resize: none;
font-family: sans-serif;
font-size: 16px;
background-color: pink;
}
.add-note {
box-sizing: border-box;
height: 200px;
border: none;
outline: none;
background: rgba(0, 0, 0, 0.15);
border-radius: 10px;
font-size: 120px;
color: rgba(0, 0, 0, 0.45);
cursor: pointer;
display: flex;
justify-content: center;
align-items: center;
transition: background 0.2s;
}
.add-note:hover {
background: rgba(0, 0, 0, 0.25);
}
HTML
<div id="app">
<button type="button" class="add-note">+</button>
</div>
JS
const notesContainer = document.getElementById('app');
const addNoteButton = notesContainer.querySelector('.add-note');
getNotes().forEach((note) => {
const noteElement = createNoteElement(note.id, note.content);
notesContainer.insertBefore(noteElement, addNoteButton);
});
addNoteButton.addEventListener('click', () => addNote());
function getNotes() {
// convert json data to native js array
return JSON.parse(localStorage.getItem('stickynotes-notes') || '[]');
}
function saveNotes(notes) {
// take in your js array and stringify to json format
localStorage.setItem('stickynotes-notes', JSON.stringify(notes));
}
function createNoteElement(id, content) {
const element = document.createElement('textarea');
element.classList.add('note');
element.value = content;
element.placeholder = 'Empty sticky note';
element.addEventListener('change', () => {
updateNote(id, element.value);
});
element.addEventListener('dblclick', () => {
const doDelete = confirm(
'Are you sure you wish to delete this sticky note?'
);
if (doDelete) {
deleteNote(id, element);
}
});
return element;
}
function addNote() {
const notes = getNotes();
const notesObject = {
id: Math.floor(Math.random() * 1000000),
content: '',
};
const noteElement = createNoteElement(notesObject.id, notesObject.content);
notesContainer.insertBefore(noteElement, addNoteButton);
notes.push(notesObject);
saveNotes(notes);
}
function updateNote(id, newContent) {
const notes = getNotes();
console.log('Updating notes: ', notes);
const targetNote = notes.filter((note) => note.id == id)[0];
targetNote.content = newContent;
saveNotes(notes);
}
function deleteNote(id, element) {
const notes = getNotes().filter((note) => note.id != id);
saveNotes(notes);
notesContainer.removeChild(element);
}
Demo
Open demo on JS Fiddle.
Link to Demo