CSS Animation Demonstrations
by Keith Rowles • 02/11/2023CSS
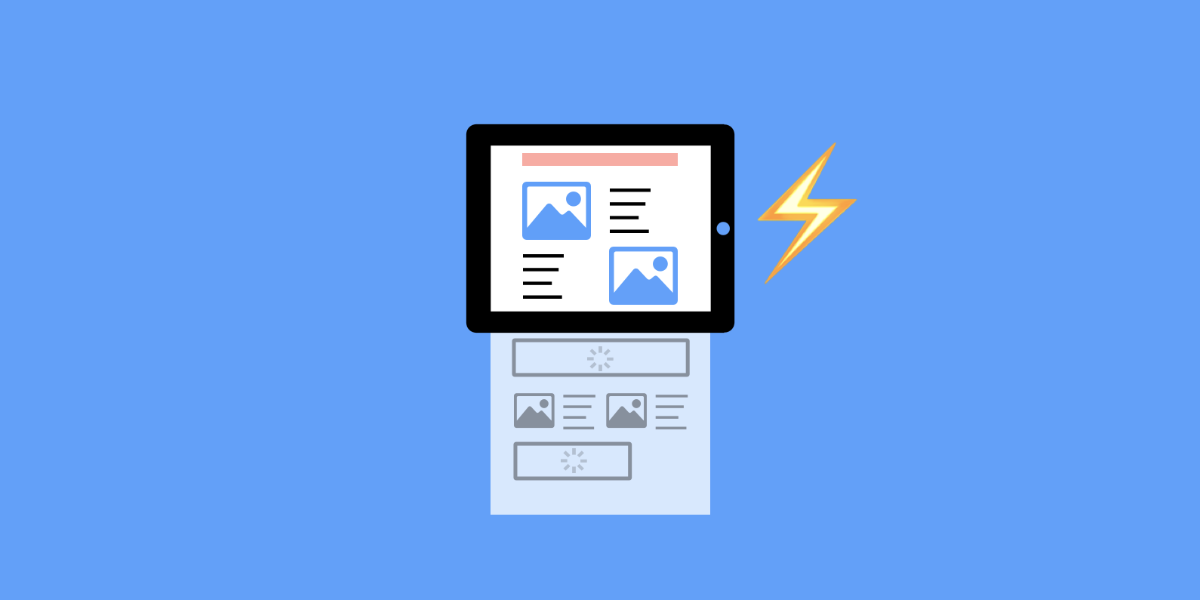
Summary
Here a few examples using CSS to create some basic animations on a web page.
Demo 1
Planes and cars moving across the screen.
HTML
<div class="sky">
<img
src="https://ragrog.com.au/code/animation//cloud.png"
alt="cloud"
class="cloud"
/>
<img
src="https://ragrog.com.au/code/animation//cloud.png"
alt="cloud"
class="cloud"
/>
<img
src="https://ragrog.com.au/code/animation//cloud.png"
alt="cloud"
class="cloud"
/>
<img
src="https://ragrog.com.au/code/animation//green-plane.png"
alt="plane"
class="plane"
/>
<img
src="https://ragrog.com.au/code/animation//green-plane.png"
alt="plane"
class="plane"
/>
<img
src="https://ragrog.com.au/code/animation//green-plane.png"
alt="plane"
class="plane"
/>
</div>
<div class="grass"></div>
<div class="road">
<div class="lines"></div>
<img
src="https://ragrog.com.au/code/animation//brown-car.png"
alt="brown car"
class="browncar"
/>
<img
src="https://ragrog.com.au/code/animation//pink-car.png"
alt="pink car"
class="pinkcar"
/>
<img
src="https://ragrog.com.au/code/animation//police-car.png"
alt="police car"
class="policecar"
/>
<img
src="https://ragrog.com.au/code/animation//sedan-car.png"
alt="sedan car"
class="sedancar"
/>
</div>
Add the CSS.
html,
body {
height: 100%;
width: 100%;
overflow: hidden;
margin: 0;
}
.grass,
.sky,
.road {
position: relative;
}
.sky {
height: 40%;
background: skyblue;
}
.grass {
height: 20%;
background: seagreen;
}
.road {
height: 40%;
background: dimgrey;
box-sizing: border-box;
border-top: 10px solid grey;
border-bottom: 10px solid grey;
width: 100%;
}
.lines {
box-sizing: border-box;
border: 3px dashed #fff;
height: 0px;
width: 100%;
position: absolute;
top: 45%;
}
/*// ELEMENTS TO ANIMATE //*/
.browncar {
position: absolute;
top: 40px;
left: 0;
animation: drive 3s both infinite linear;
}
.pinkcar {
position: absolute;
top: 100px;
left: 0;
animation-name: drive;
animation-duration: 5s;
animation-fill-mode: both;
animation-iteration-count: infinite;
animation-direction: normal;
animation-timing-function: linear;
}
.policecar {
position: absolute;
top: 180px;
left: 0;
animation-name: drive;
animation-duration: 7s;
animation-fill-mode: both;
animation-iteration-count: infinite;
animation-direction: normal;
animation-timing-function: ease-out;
}
.sedancar {
position: absolute;
top: 250px;
left: 0;
animation-name: drive;
animation-duration: 3s;
animation-fill-mode: both;
animation-iteration-count: infinite;
animation-direction: normal;
animation-timing-function: ease-in;
}
.cloud {
position: absolute;
}
.cloud:nth-child(1) {
width: 100px;
top: 120px;
opacity: 0.5;
animation: wind 50s linear infinite reverse;
}
.cloud:nth-child(2) {
width: 200px;
top: 0;
animation: wind 30s linear infinite reverse;
}
.cloud:nth-child(3) {
width: 150px;
top: 200;
opacity: 0.7;
animation-name: wind;
animation-duration: 45s;
animation-fill-mode: both;
animation-iteration-count: infinite;
animation-direction: reverse;
animation-timing-function: linear;
animation-delay: 10s;
}
.plane {
position: absolute;
}
.plane:nth-child(4) {
width: 50px;
top: 100px;
animation: fly 10s linear infinite reverse;
}
.plane:nth-child(5) {
width: 70px;
top: 200px;
animation: fly 20s linear infinite reverse, flycrazy 0.3s 1s ease;
filter: hue-rotate(45deg);
}
.plane:nth-child(6) {
width: 200px;
top: 250px;
filter: hue-rotate(90deg);
animation-name: flycrazy;
animation-duration: 5s;
animation-fill-mode: both;
animation-iteration-count: infinite;
animation-direction: reverse;
animation-timing-function: linear;
animation-delay: 10s;
}
/*// KEYFRAMES //*/
@keyframes drive {
from {
transform: translateX(-200px);
}
to {
transform: translateX(2000px);
}
}
@keyframes fly {
from {
transform: translateX(-200px);
}
to {
transform: translateX(2000px);
}
}
@keyframes wind {
from {
left: -300px;
}
to {
left: 100%;
}
}
@keyframes flycrazy {
0% {
top: 200px;
left: -300px;
}
50% {
top: 150px;
}
100% {
top: 200px;
left: 100%;
}
}
Link to Demo 1 on Codepen.
Link to DemoMake sure to open the code panels on the left so the results page has maximum height.
Demo 2
Pick a box - flipping cards.
Link to Demo 2 on Codepen.
Link to Demo