Gatsby React Site Generator Tutorial
by Keith Rowles • 09/08/2023Framework
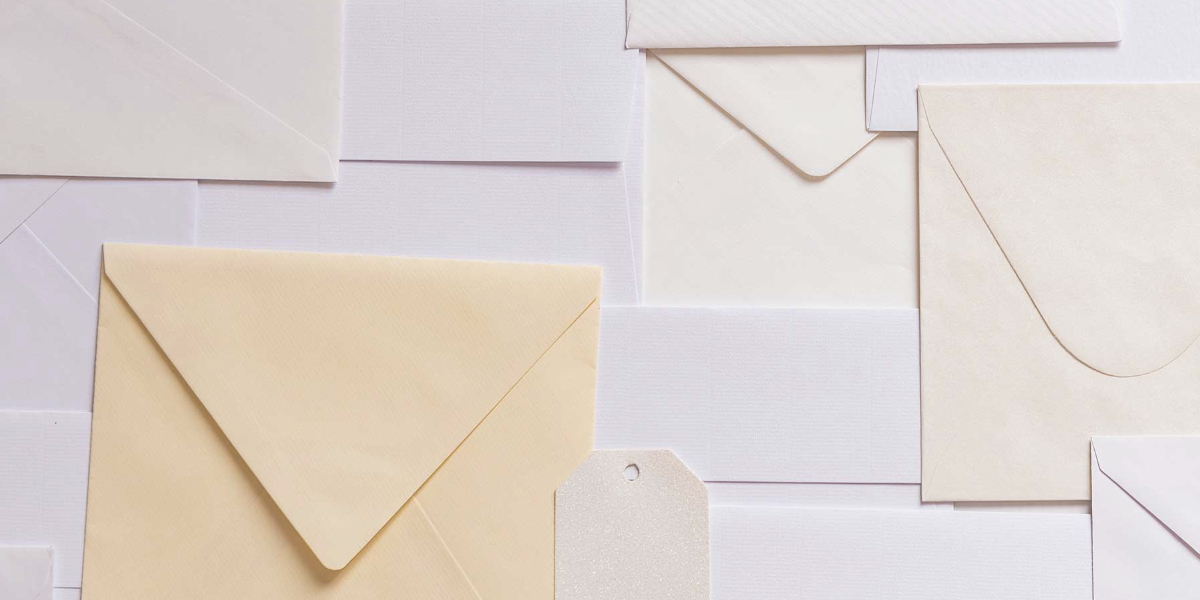
Summary
Completed the Net Ninja Gatsby JS tutorial to create a blog website then deployed to Netlify.
The project uses markdown files to create post content.
Also incorporates Gatsby plugins:
- Image
- Sharp
Tech and Tools
- CSS
- HTML
- JavaScript
- GitHub
- Netlify
- Gatsby JS
Dependencies
"dependencies": {
"gatsby": "^4.24.0",
"gatsby-plugin-image": "^2.24.0",
"gatsby-plugin-sharp": "^4.24.0",
"gatsby-source-filesystem": "^4.24.0",
"gatsby-transformer-remark": "^5.24.0",
"gatsby-transformer-sharp": "^4.24.0",
"react": "^18.1.0",
"react-dom": "^18.1.0"
}
Sample Code
index.js
import { graphql, Link } from 'gatsby';
import React from 'react';
import Layout from '../components/Layout';
import * as style from '../styles/home.module.css';
import { GatsbyImage, getImage } from 'gatsby-plugin-image';
export default function Home({ data }) {
console.log(data);
const image = getImage(data.file.childImageSharp.gatsbyImageData);
return (
<Layout>
<section className={style.header}>
<div>
<h2>Design</h2>
<h3>Develop and Deploy</h3>
<p>UX designer and web developer based in Brisbane</p>
<Link className={style.btn} to="/projects">
My Portfolio Projects
</Link>
</div>
<GatsbyImage image={image} alt="Banner" />
</section>
</Layout>
);
}
// use a fragment in query
export const query = graphql`
query Banner {
file(relativePath: { eq: "banner.png" }) {
childImageSharp {
gatsbyImageData(
layout: FULL_WIDTH
placeholder: BLURRED
formats: [AUTO, WEBP]
)
}
}
}
`;
projects.js
import { graphql, Link } from 'gatsby';
import React from 'react';
import Layout from '../../components/Layout';
import * as style from '../../styles/projects.module.css';
import { GatsbyImage, getImage } from 'gatsby-plugin-image';
export default function Projects({ data }) {
console.log(data);
// projects array
const projects = data.projects.nodes;
const contact = data.contact.siteMetadata.contact;
return (
<Layout>
<div className={style.portfolio}>
<h2>Portfolio</h2>
<h3>Projects and Websites I have created.</h3>
<div className={style.projects}>
{projects.map((project) => (
<Link to={'/projects/' + project.frontmatter.slug} key={project.id}>
<div>
<GatsbyImage
image={getImage(
project.frontmatter.thumb.childImageSharp.gatsbyImageData
)}
alt="Banner"
/>
<h3>{project.frontmatter.title}</h3>
<p>{project.frontmatter.stack}</p>
</div>
</Link>
))}
</div>
<p>Like what you see? email me at {contact} for a quote.</p>
</div>
</Layout>
);
}
// export page query
export const query = graphql`
query ProjectsPage {
projects: allMarkdownRemark(
sort: { fields: frontmatter___date, order: DESC }
) {
nodes {
frontmatter {
title
stack
slug
date
thumb {
childImageSharp {
gatsbyImageData(
layout: FULL_WIDTH
placeholder: BLURRED
formats: [AUTO, WEBP]
)
}
}
}
id
}
}
contact: site {
siteMetadata {
contact
}
}
}
`;
Demo
Link to demo on Netlify.
Link to DemoGitHub Repo
Open GitHub Repository.
Link to Repo