React Material UI Products UI
by Keith Rowles • 15/12/2023React
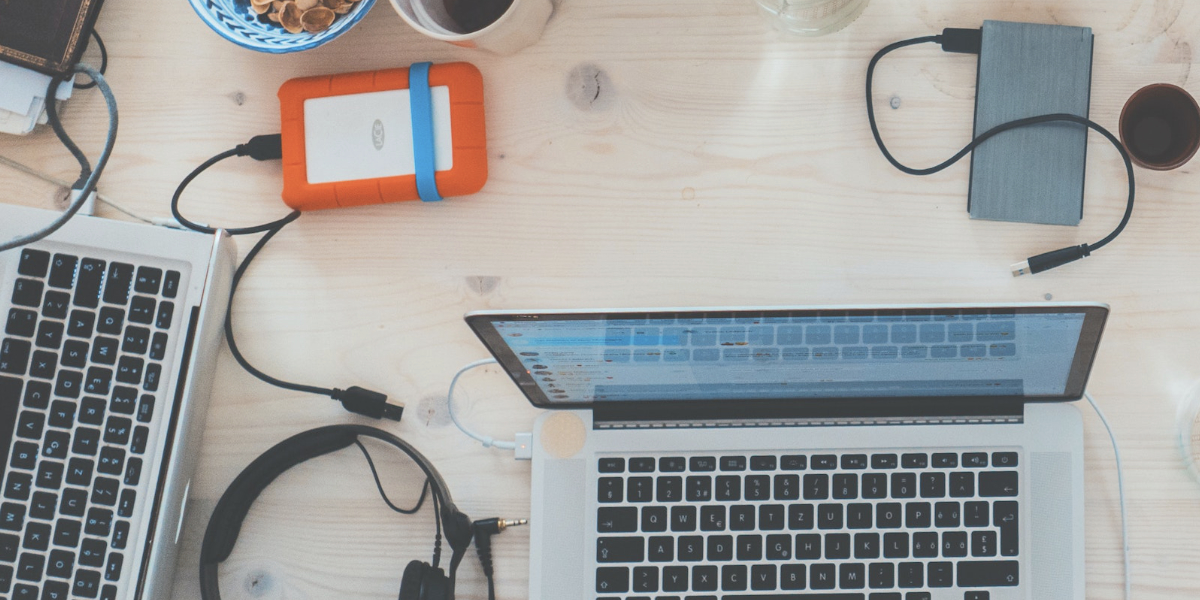
Summary
Created a products dashboard with Material UI, a component library with production ready components.
The main purpose of this demo is to use some of the components available in the library.
Note: The dashboard lists out some products with a title, brand, description, category and image.
Material UI Components used in the build:
- Button
- Radio Group
- Text Field
- Avatar
- Chip
- Material Icons
- Typography
- Alert
- App Bar
- Card
- Box
- Container
Tech and Tools
- JavaScript
- CSS
- HTML
- JSX
- React
- MUI Components
- cPanel
- API
Sample Code
Projects.jsx
import { useState, useEffect } from 'react';
import Container from '@mui/material/Container';
import Masonry from 'react-masonry-css';
import ProductCard from '../components/ProductCard';
export default function Products() {
const [products, setProducts] = useState([]);
useEffect(() => {
fetch('https://dummyjson.com/products')
.then((res) => res.json())
.then((data) => setProducts(data.products));
}, []);
const handleDelete = async (id) => {
await fetch('https://dummyjson.com/products/' + id, {
method: 'DELETE',
});
const newProducts = products.filter((product) => product.id !== id);
setProducts(newProducts);
};
const breakpointColumnsObj = {
default: 3,
1100: 2,
700: 1,
};
return (
<Container>
<Masonry
breakpointCols={breakpointColumnsObj}
className="my-masonry-grid"
columnClassName="my-masonry-grid_column"
>
{products.map((product) => (
<div key={product.id}>
<ProductCard product={product} handleDelete={handleDelete} />
</div>
))}
</Masonry>
</Container>
);
}
ProductCard.jsx
/* eslint-disable react/prop-types */
import Card from '@mui/material/Card';
import CardHeader from '@mui/material/CardHeader';
import Avatar from '@mui/material/Avatar';
import IconButton from '@mui/material/IconButton';
import CardContent from '@mui/material/CardContent';
import CardMedia from '@mui/material/CardMedia';
import Chip from '@mui/material/Chip';
import { DeleteOutlined } from '@mui/icons-material';
import { Typography } from '@mui/material';
import {
green,
blue,
pink,
yellow,
red,
purple,
amber,
cyan,
orange,
brown,
} from '@mui/material/colors';
const ProductCard = ({ product, handleDelete }) => {
const ylow = yellow[700];
const grn = green[500];
const bl = blue[500];
const pnk = pink[500];
const rd = red[500];
const purp = purple[400];
const amb = amber[500];
const cyn = cyan[500];
const orng = orange[500];
const brn = brown[500];
return (
<div>
<Card
elevation={3}
sx={product.brand === 'Apple' ? { border: '1px solid red' } : null}
>
<CardHeader
style={{ textAlign: 'left' }}
action={
<IconButton onClick={() => handleDelete(product.id)}>
<DeleteOutlined />
</IconButton>
}
title={product.title}
subheader={product.brand}
avatar={
<Avatar
sx={{
backgroundColor:
product.brand === 'Apple'
? ylow
: product.brand === 'Samsung'
? grn
: product.brand === 'OPPO'
? pnk
: product.brand === 'Huawei'
? rd
: product.brand === 'Microsoft Surface'
? purp
: product.brand === 'Infinix'
? amb
: product.brand === 'Golden'
? cyn
: product.brand === 'HP Pavilion'
? orng
: product.brand === 'Royal_Mirage'
? brn
: bl,
}}
>
{product.brand[0].toUpperCase()}
</Avatar>
}
/>
<CardMedia
component="img"
alt={product.title}
height="150"
image={product.thumbnail}
/>
<CardContent>
<Typography
variant="body2"
color="text.secondary"
align="left"
mb={2}
>
{product.description}
</Typography>
<Chip label={product.category} color="primary" size="small" />
</CardContent>
</Card>
</div>
);
};
export default ProductCard;
Demo
Open demo on a sub domain on my shared hosting service (cPanel).
Link to DemoMUI
Here is the link to MUI website.
Link to MUIAPI
The fake product list come from the dummyJSON website.
Here is the link to JSON data.
Link to API