React Styled Components Landing Page
by Keith Rowles • 16/12/2023React
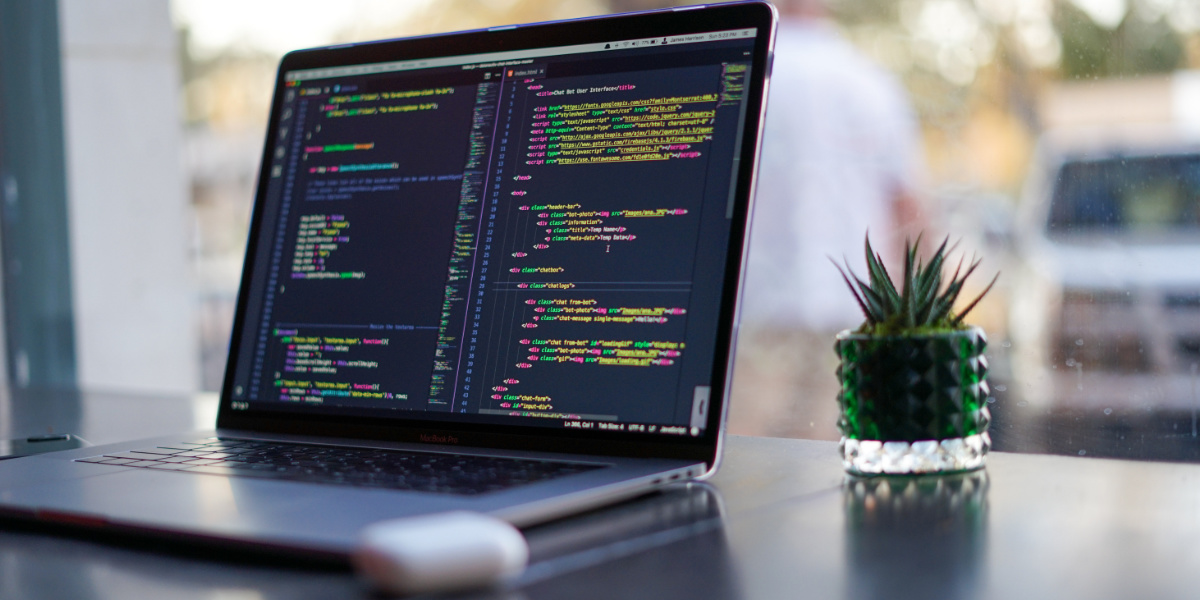
Summary
A website landing page with a header, hero section, cards and footer built with React and Styled Components.
Project from Traversy Media and Front End Mentors.
Tech and Tools
- JavaScript
- CSS
- HTML
- React
- Styled Components
- cPanel
Sample Code
Social icons component.
Socialicons.js
import { FaTwitter, FaFacebook, FaLinkedin } from 'react-icons/fa';
import { StyledSocialIcons } from './styles/Socialicons.styled';
export default function Socialicons() {
return (
<StyledSocialIcons>
<li>
<a href="https://twitter.com" target="_blank" rel="noreferrer">
<FaTwitter />
</a>
</li>
<li>
<a href="https://facebook.com" target="_blank" rel="noreferrer">
<FaFacebook />
</a>
</li>
<li>
<a href="https://linkedin.com" target="_blank" rel="noreferrer">
<FaLinkedin />
</a>
</li>
</StyledSocialIcons>
);
}
Socialicons.styled.js
import styled from 'styled-components';
export const StyledSocialIcons = styled.div`
display: flex;
align-items: center;
justify-content: center;
li {
list-style: none;
}
a {
border: 1px solid #fff;
border-radius: 50%;
color: #fff;
display: inline-flex;
align-items: center;
justify-content: center;
margin-right: 10px;
height: 40px;
width: 40px;
text-decoration: none;
}
`;
Card.js
Card component.
import { StyledCard } from './styles/Card.styled';
export default function Card({ item: { id, title, body, image } }) {
return (
<StyledCard layout={id % 2 === 0 && 'row-reverse'}>
<div>
<h2>{title}</h2>
<p>{body}</p>
</div>
<div>
<img src={`./images/${image}`} alt="" />
</div>
</StyledCard>
);
}
Card.styled.js
import styled from 'styled-components';
export const StyledCard = styled.div`
display: flex;
align-items: center;
background-color: #fff;
border-radius: 15px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.15);
margin: 40px 0;
padding: 60px;
flex-direction: ${({ layout }) => layout || 'row'};
img {
width: 80%;
}
& > div {
flex: 1;
}
@media (max-width: ${({ theme }) => theme.mobile}) {
flex-direction: column;
}
`;
Demo
Open demo on my shared hosting service (cPanel).
Link to DemoStyled Components
Here is the link to the styled components website.
Link to MUI