Standard For Loop and ForEach Loop
by Keith Rowles • 05/12/2022JavaScript
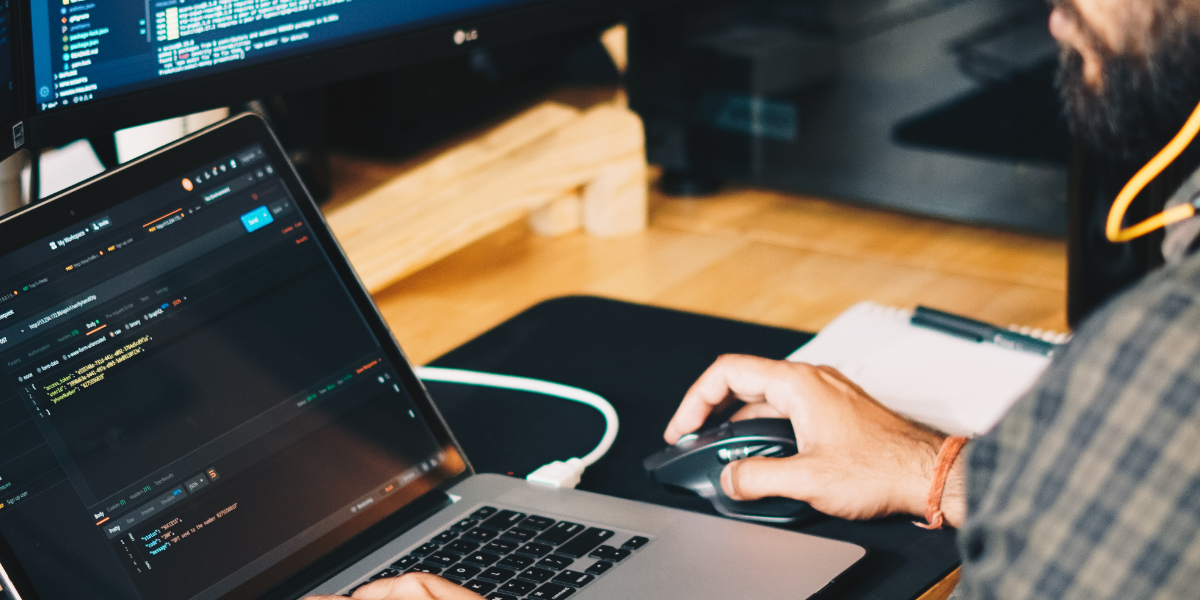
Summary
A few examples using the standard for loop and the ForEach loop to output some arrays.
There is a link to the demo at the bottom of the page.
Tech
- HTML
- JavaScript
HTML
Add some containers in your web page to display the output.
<div class="container">
<h2>Fruit Output</h2>
<div id="outputOne" class="alert alert-info"></div>
<hr />
<h2>Number Output</h2>
<div id="outputTwo" class="alert alert-info"></div>
<hr />
<h2>Month Output</h2>
<div id="outputThree" class="alert alert-info"></div>
<hr />
<h2>Dinner Output</h2>
<div id="outputFour" class="alert alert-info"></div>
<hr />
<h2>Fast Food Output</h2>
<div id="outputFive" class="alert alert-info list-unstyled"></div>
<hr />
<h2>Veges Output</h2>
<div id="outputSix" class="alert alert-info"></div>
<hr />
<h2>Company Output</h2>
<div id="outputSeven" class="alert alert-info list-unstyled"></div>
</div>
JS
Here are the arrays:
const companies = [
{
name: 'Company One',
category: 'Finance',
start: 1981,
end: 2004,
},
{
name: 'Company Two',
category: 'Retail',
start: 1992,
end: 2008,
},
{
name: 'Company Three',
category: 'Auto',
start: 1999,
end: 2007,
},
{
name: 'Company Four',
category: 'Retail',
start: 1989,
end: 2010,
},
{
name: 'Company Five',
category: 'Technology',
start: 2009,
end: 2014,
},
{
name: 'Company Six',
category: 'Finance',
start: 1987,
end: 2010,
},
{
name: 'Company Seven',
category: 'Auto',
start: 1986,
end: 1996,
},
{
name: 'Company Eight',
category: 'Technology',
start: 2011,
end: 2016,
},
{
name: 'Company Nine',
category: 'Retail',
start: 1981,
end: 1989,
},
];
const fruits = [
'apple',
'orange',
'cherry',
'pear',
'banana',
'melon',
'strawberry',
];
const numbers = [65, 44, 12, 4];
const months = ['January', 'February', 'March', 'April'];
const dinner = [
'meat pie',
'spagetti',
'marinara',
'baked beans',
'sausage roll',
];
const fastfooditems = [
{ id: '🍔', name: 'Super Burger', price: 399 },
{ id: '🍟', name: 'Jumbo Fries', price: 199 },
{ id: '🥤', name: 'Big Slurp', price: 299 },
];
var veggies = ['Potato', 'Spinach', 'Capsicum', 'Pumpkin', 'Carrots'];
Code To Display Fruits Arrays
let text = '';
let fruittext = '';
// for loop
for (i = 0; i < fruits.length; i++) {
fruittext += [i] + ': ' + fruits[i] + '<br>';
}
document.getElementById('outputOneTwo').innerHTML = fruittext;
// forEach
fruits.forEach(outputMyArray);
document.getElementById('outputOne').innerHTML = text;
function outputMyArray(item, index) {
text += index + ': ' + item + '<br>';
}
Code To Display Numbers Array
let sum = 0;
numbers.forEach(sumMyArray);
document.getElementById('outputTwo').innerText = sum;
function sumMyArray(item) {
sum += item;
}
let nums = '';
for (let x in numbers) {
nums += numbers[x] + '<br>';
}
document.getElementById('outputTwoTwo').innerHTML = nums;
Code To Display Months Array
let month = '';
months.forEach(function (item, index, arr) {
month += index + ' : ' + item + ' : ' + arr + '<br>';
});
document.getElementById('outputThree').innerHTML = month;
Code To Display Dinner Array
let mydinner = '';
dinner.forEach((item, index, array) => {
mydinner += `${item} is at index ${index} in array: [${array}]` + '<br><br>';
});
document.getElementById('outputFour').innerHTML = mydinner;
Code To Display Fast Food Array
const burgerBox = document.querySelector('#outputFive');
fastfooditems.forEach((item) => {
burgerBox.innerHTML += `
<li>
${item.id} ${item.name} - ${(item.price / 100).toFixed(2)}
</li>
`;
});
Code To Display Veggies Array
veggies.forEach(listMyVeges);
function listMyVeges(itemName, index) {
document.getElementById('outputSix').innerHTML +=
index + ': ' + itemName + '<br>';
}
Code To Display Company Array
const listOfCompanies = document.querySelector('#outputSeven');
companies.forEach((item) => {
listOfCompanies.innerHTML += `
<li>
${item.name} ${item.category} - ${item.start} : ${item.end}
</li>
`;
});
Demo
Open demo on Code Pen. (Clicking the link will open up a new window.)
Link to Demo