React JS and SyncFusion Dashboard
by Keith Rowles • 08/01/2024React
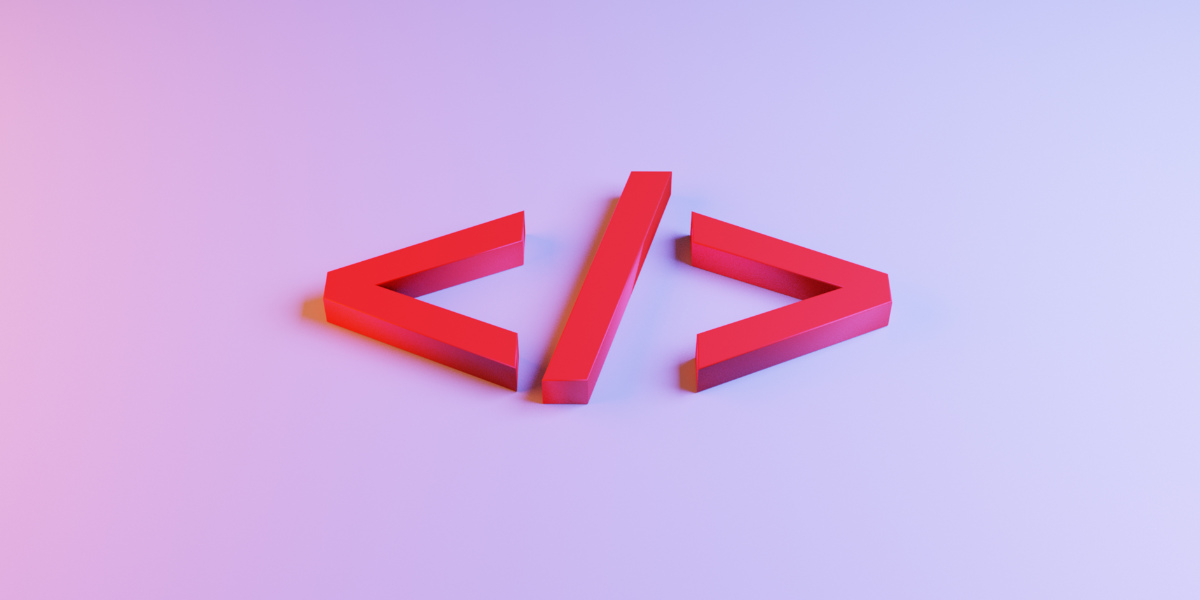
Summary
A React JS dashboard application built with SyncFusion, a component library with production ready components.
The main purpose of this demo is to use some of the components available in the SyncFusion library.
SyncFusion components used in the build include:
- Calendar
- Color Picker
- Grid
- Editor
- Kanban
- Button
- Tool Tip
- Charts
Tech and Tools
- CSS
- HTML
- JavaScript
- JSX
- React
- SyncFusion UI Components
- Netlify
- Tailwind CSS
- Post CSS
Sample Code
kanban.jsx
import React from 'react';
import {
KanbanComponent,
ColumnsDirective,
ColumnDirective,
} from '@syncfusion/ej2-react-kanban';
import { kanbanData, kanbanGrid } from '../data/dummy';
import { Header } from '../components';
const Kanban = () => {
return (
<div className="m-2 md:m-10 mt-24 p-2 md:p-10 bg-white rounded-3xl">
<Header title="Kanban" category="App" />
<KanbanComponent
id="kanban"
dataSource={kanbanData}
cardSettings={{ contentField: 'Summary', headerField: 'Id' }}
keyField="Status"
>
<ColumnsDirective>
{kanbanGrid.map((item, index) => (
<ColumnDirective key={index} {...item} />
))}
</ColumnsDirective>
</KanbanComponent>
</div>
);
};
export default Kanban;
editor.jsx
import React from 'react';
import {
HtmlEditor,
Image,
Inject,
Link,
QuickToolbar,
RichTextEditorComponent,
Toolbar,
} from '@syncfusion/ej2-react-richtexteditor';
import { EditorData } from '../data/dummy';
import { Header } from '../components';
const Editor = () => {
return (
<div className="m-2 md:m-10 mt-24 p-2 md:p-10 bg-white rounded-3xl">
<Header title="Editor" category="App" />
<RichTextEditorComponent>
<EditorData />
<Inject services={[HtmlEditor, Toolbar, Image, Link, QuickToolbar]} />
</RichTextEditorComponent>
</div>
);
};
export default Editor;
Demo
Open demo on a Netlify.
This is a manual deploy.
Link to Demo